How to easily add a Sql database in Xamarin Forms
Every good app needs to use a database. This database can be local (present in the app itself), or it can be remote (saved somewhere on the internet). The third option (fairly common) is to use both and then use a synchronisation mechanism between the two databases. In the case of a local database, the best possible option for our apps is to use an SQL database and use the SQLite library. This library can be used on mobile phones and PCs and is present on a multitude of different applications.
Use SQLite in Xamarin
In theory (I tell you later a better way) to add and use a SQL database in Xamarin.Forms you need to install the “sqlite-net-pcl” library in your projects (.netstandard, Android, iOS, UWP) and then create Dependencies Services (one for each platform).
The whole procedure is not particularly complicated, but it takes about half an hour. If you multiply half an hour for each project you are going to create, you will see that the amount of time lost becomes considerable.
After so many projects and many lost hours I decided to create and make available for everyone my library based on “sqlite-net-pcl“.
My library is called MtSql and allows you to add a SQL database to Xamarin apps in few seconds and in an extremely easy way. Going from 30 minutes to a few seconds is no small feat so I’ll explain how.
MTSQL
My library works with Xamarin and Windows, so you can use it in practically every project where you need to use a database. As this is a blog about Xamarin, I show you how it works on this platform.
Here are the steps to use a SQLite database in your Xamarin.Forms project.
- Right click on your solution and select Manage Nuget Packages for Solution
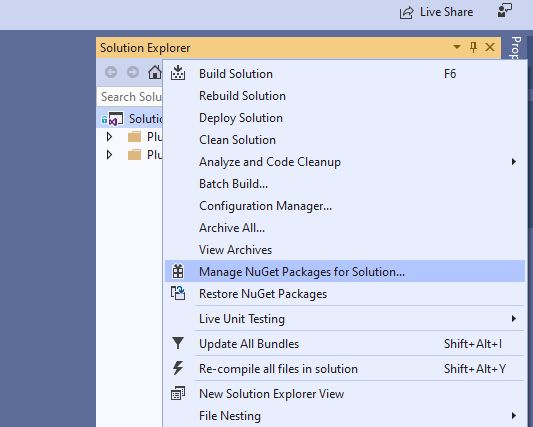
- Search for “MarcTron” (so you can see my other plugins too 🙂 ) or directly MTSql. Install MarcTron.Sqlite in your projects (.netstandard, Android, iOS, UWP).
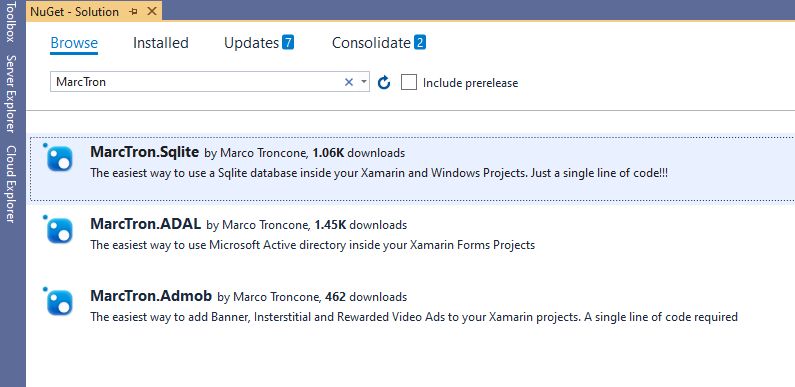
- Finished!!!! You have nothing else to do, right now you are ready to use a SQL database inside your app. Time taken: few seconds 🙂
How to use MTSQL
Now that the library is installed in your solution, we need to tell our app which database to use, so we need to establish a connection with the database. To do this we have several possibilities:
using MarcTron.Plugin;
...
SQLiteConnection conn = MtSql.Current.GetConnection("yourdbname.db3");
in this case we have established a connection with the “yourdbname.db3” database. If we want to create an asynchronous connection, we can instead write:
SQLiteAsyncConnection conn = MtSql.Current.GetConnectionAsync("yourdbname.db3");
Since version 1.2 of MTSql I have created an additional Helper to make everything even easier, so to establish the connection with your database, you can write:
MtSql.Helper.Initialize("yourdbname.db3");
PLEASE NOTE: You must always initialise the database with one of the three previous methods before using it.
In my library, to simplify your life, I’ve created a basic template for the tables in your database. This model is called BaseModel (I write it here just to show it to you):
public class BaseModel
{
[PrimaryKey]
public string Id { get; set; }
public DateTime CreatedAt { get; set; }
public DateTime UpdatedAt { get; set; }
}
Using it is very simple. For example, if you have a table called User with a Name field, you can write the class that defines it as:
public class User : BaseModel
{
public string Name{ get; set; }
}
Doing so your table will have not only the Name field but also a primary Id key and two CreatedAt, UpdatedAt dates, which specify when the data has been created and modified (very useful if you want to sort your tables by creation or update date).
The helper I created has inside a method called Save. This method will deal not only with inserting or updating a record within your tables but also creating a GUID for you (if you have not already created it) and updating the value of CreatedAt and UpdatedAt. FANTASTIC!
PLEASE NOTE: The Save method inserts an object if its primary key value is not present in the table while updating it if its value is already in the table.
You don’t have to use the BaseModel class but I suggest you to do so and now I’ll show you why.
An Example
Now let’s see a quick example to initialise the database, insert an object and then update it:
MtSql.Helper.Initialize("MyDatabase.db3");
MtSql.Helper.CreateTable<User>();
User user = new User() {Name = "Marco"};
MtSql.Helper.Save(user);
user .Name = "Marco Troncone";
MtSql.Helper.Save(user);
In the first line we have initialised the database (Don’t forget it, it is fundamental to do it).
We then created a User table using the User class written above. At this point we created a user and gave him the name Marco.
When we call the method
MtSql.Helper.Save(user);
since user does not have an Id, the Save method will create one automatically (if you want you can assign one yourself) and will add the user to the table, setting CreatedAt and UpdatedAt to the current time.
At this point we try to change the user name and save the change:
user.Name = "Marco Troncone";
MtSql.Helper.Save(user);
This time user has already an Id and this Id is already present in the table, this time the Save method will not add a new object to the table but will update the current object, automatically updating UpdatedAt. Easy right?
Thanks to the MTSql plugin, in exactly 6 lines of code we have initialised our database, created a table, added an object and modified an object.
Other methods inside the Helper
In the previous example, we’ve seen some helper methods (Initialise, CreateTable, Save), let’s see what the other methods we have:
//To insert a single item inside the table
Insert<TType>(TType item);
//To insert multiple items inside the table
InsertAll<TType>(IEnumerable<TType> items);
//To update an item inside the table
Update<TType>(TType item);
//To find an item inside the table
FirstOrDefault<TType>(Expression<Func<TType, bool>> expression);
//To get from the table the item with Id itemId
GetFromId<TType>(string itemId);
//To get from the table the items with parameter quals to value
GetBy<TType>(string parameter, object value);
//To get all the items from the table
GetAll<TType>();
//To delete the item from the table
Delete<TType>(TType item);
//To delete ALL the items from the table. WARNING!!!
DeleteAll<TType>();
//To see if an item exists
Exists<TType>(string itemId);
//To count the number of items in a table
Count<TType>();
The Helper I’ve created has all the main methods to work on your database. If some methods are not available, you can directly access the database (and therefore all its methods) using the command:
MtSql.Helper.Conn
this is a direct link to the database connection and from here you can find all the supported methods.
Conclusions
Creating a database could be a complicated operation but thanks to the MTSql library, creating a database for our Xamarin projects. Forms is incredibly easy. Install the library from Nuget and in a few seconds and a few lines of code, you have a database ready to be used in your Android, iOS and UWP projects.
Do you have any doubts or suggestions? Add a comment below, and if the article and the library were helpful to you, share them.
December 6, 2021 @ 8:43 pm
Marco, In the intro you mention the ‘third option’: syncronizing between a remote and local database. Does MTSql help with this scenario? Do you have a tutorial for this operation?
December 6, 2021 @ 8:57 pm
Hi,
This library doesn’t provide any sync functionalities.
It provides a basemodel and some helper methods that might be a base to help with it but there is nothing specific.
April 14, 2020 @ 7:37 pm
The sentence ” MtSql.Helper.Save(user);” simply don’t work.
Trows this error:
Severity Code Description Project File Line Suppression State
Error CS0311 The type ‘EFC2.User’ cannot be used as type parameter ‘TType’ in the generic type or method ‘MtSqlHelper.Save(TType)’. There is no implicit reference conversion from ‘EFC2.User’ to ‘MarcTron.Plugin.Models.BaseModel’. EFC2 C:\Users\Luis\source\repos\EFC2\EFC2\MainPage.xaml.cs 28 Active
Apart from that, in the Readme.txt file, the references are written as “MTSql”. Should be: “MtSql” (note the letter T)
¿Any suggestion about what to do?
Thank you
April 14, 2020 @ 7:16 pm
Hi Luis,
Yes, to use the generic Save, your model should inherit from BaseModel.
Something like:
Public class User : BaseModel
If you do it, you’ll have an index and the the fields CreatedAt and UpdatedAt and you will be able to use the Save method!
October 14, 2019 @ 4:48 pm
Hola Marcos te hago una consulta como hago para ver los datos en un gris soy nuevo en xAMARÃN y en el mundo de la programación.
Desde ya muchas gracias